What is JSON?
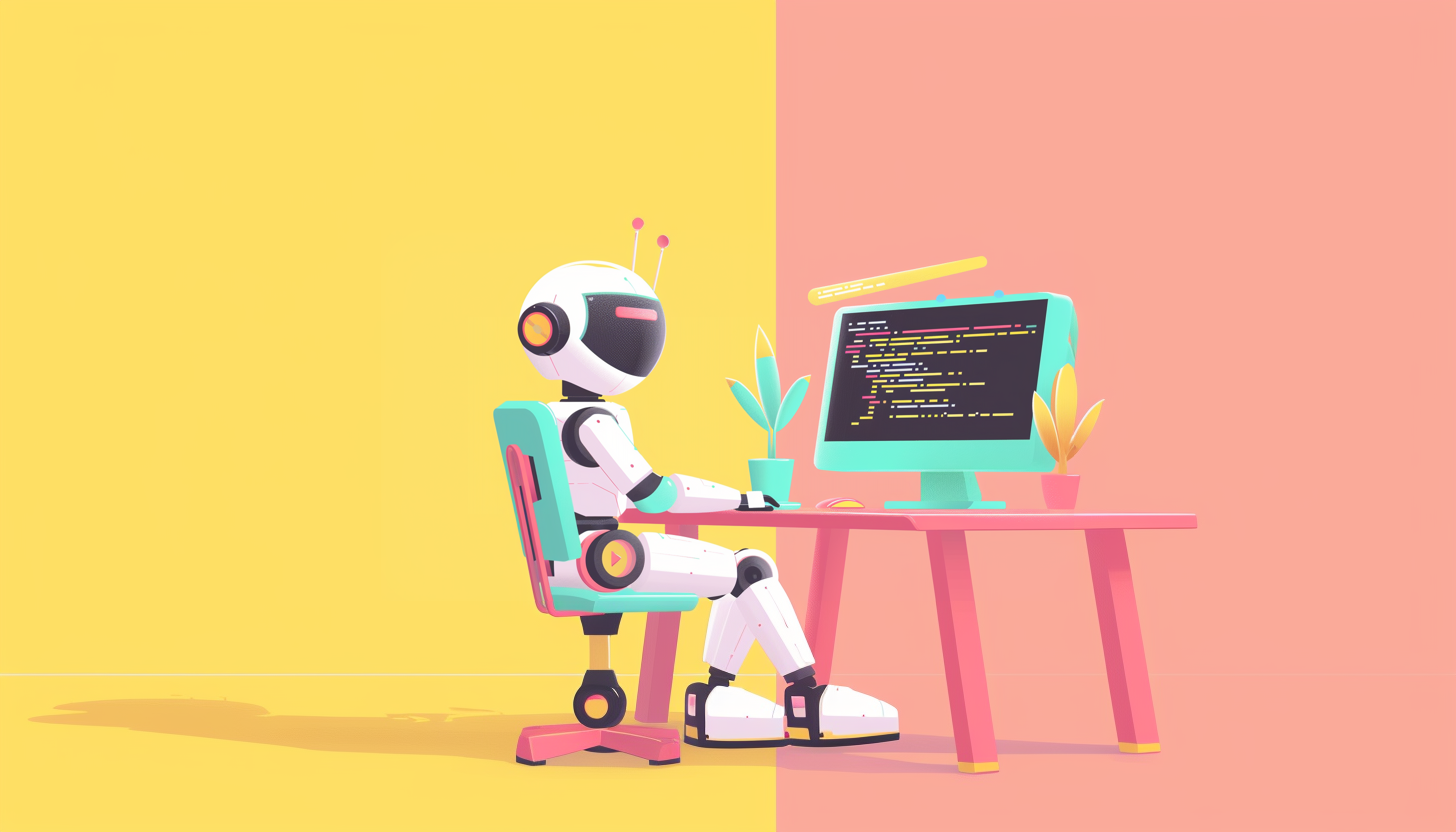
The internet runs on data. For applications and servers to communicate effectively, they need a common language that's both easy for computers to parse and somewhat human-readable. This is where JSON (JavaScript Object Notation) enters the scene. JSON is a lightweight data format, now the lingua franca of web data exchange.
Its popularity stems from several advantages over other formats:
- Easy to Read: JSON resembles programming code but is less complex, making it clear for humans and computers alike.
- Works across languages and frameworks: JSON's independence from specific programming languages enhances flexibility, facilitating data sharing between diverse applications.
- Developer-Friendly: JSON's simplicity saves programmers time and effort in data exchange applications.
In short, JSON makes data sharing on the web simple and efficient. It's like a universal translator for applications, allowing them to communicate and exchange information smoothly.
JSON Payload Basic Structure
To get a better idea, let's jump right into some sample JSON data. Let's say you make an API request to fetch weather data for a city. A typical JSON response might look like this:
{
"city": "London",
"temperature": 15,
"unit": "Celsius",
"description": "Cloudy",
"isRaining": false, // Boolean (false)
"feelsLike": null, // Null value
"forecast": [
{ "day": "Monday", "temp": 16 },
{ "day": "Tuesday", "temp": 18 }
]
}
Let's breakdown what this data represents, and the properties of JSON being used here:
- Objects: The outermost structure represents an object, enclosed by curly braces
{}
. An object is an unordered collection of key-value pairs. - Key-Value Pairs: Each line within the object contains a key, a colon (:), and a value. For instance, "city" is a key, and "London" is its associated value.
- Data Types for Values: JSON supports various data types, including strings (e.g., "city"), numbers (e.g., "temperature", with a value of 15), arrays (e.g., "forecast"), and objects themselves (each element of the forecast array is itself an object).
- Arrays: Arrays are ordered sequences of values, represented by square brackets []. In this example, "forecast" is an array containing two objects.
- Nested Objects: JSON objects can be nested within other objects. The "forecast" array contains two nested objects, each representing a day's weather forecast.
- Comments: JSON does not support comments. This is because JSON is a data interchange format, and comments are not considered data. However, some JSON parsers might ignore comments, so you might see them in JSON files used for configuration or documentation purposes, but generally not in API responses.
We also have primitive types, which are raw data types that cannot be broken down further. Here's a breakdown of the primitive data types used in this example:
- Strings: Represent text data, enclosed in double quotes ("). Example: "city", "description"
- Numbers: Represent numeric values, either integers or floating-point numbers. Example: "temperature" (whose value is
15
) - Booleans: Represent logical values, either
true
orfalse
. Example: "isRaining" (set to indicate it's not raining) - Null: Represents an empty or missing value. Example: "feelsLike" (indicating the "feelsLike" temperature is unknown or not set)
Note that the initial JSON structure doesn’t need to be an object. It could also be a primitive data type like a string, number, boolean, or an array. However, in most cases, the top-level structure is an object, as it allows for more complex data structures to be represented.
Reading JSON from HTTP Responses
In real world applications, In real-world applications, JSON serves as the data interchange format between systems. Most notably, this is used as the request and response format in HTTP exchanges.
If a client web page sends an HTTP request to a server application endpoint, the server responds with a JSON payload.
Continuing on with our previous example, imagine a client web page wants to retrieve the current weather for London. Let's examine the process components:
Endpoints: The server application has an endpoint specifically designed to handle weather data requests. Let's call it /weather
for this example. This endpoint is represented as a URL on the server that the client can call.
Client Request:
- Method: The client (web page or application) initiates an HTTP request using the
GET
method. This method signifies the client is requesting data from the server. - URL: The URL of the request specifies the server's address and the endpoint. In this case, it would be something like
https://www.example.com/weather
.
Server Response:
- Status Code: Upon receiving the request, the server processes it and send back an HTTP response. The response will include a status code indicating the outcome. A successful response would have a status code of
200 OK
. - Headers: The response headers contain information about the response data, such as the content type. For JSON responses, the server should include a
Content-Type: application/json
HTTP header to tell the client the response body contains JSON data. - JSON Response Body: The most crucial part of the response is the body, which in this example will be formatted in JSON. This would look similar to the JSON data we discussed earlier, containing the weather details for London.
Client Action:
The client's web page will receive the response from the server. It can then parse the JSON data and use the information (temperature, description, forecast) to display the weather details for London to the user.
Remember: This is a simplified example. Real-world HTTP requests and responses can involve additional complexities like authentication tokens, authorization checks, and error handling.
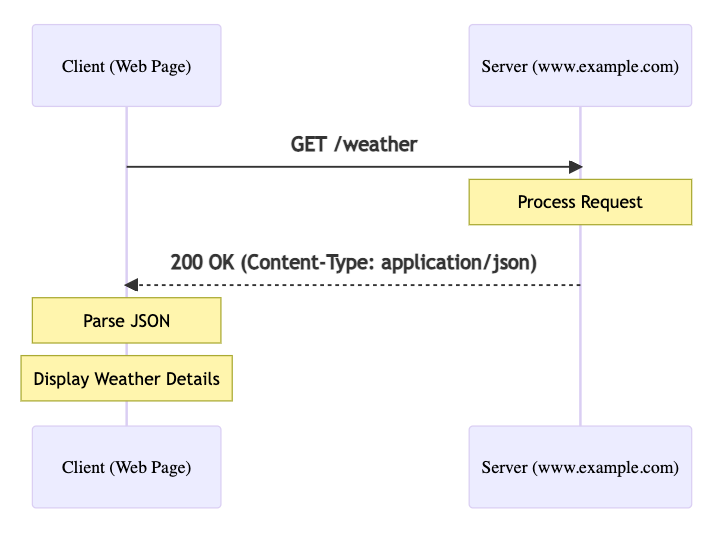
Processing JSON Data
Let's see how the client application can process the JSON data received from the server.
In most cases, we receive the raw JSON response as a string. To work with this data in JavaScript, we need to convert it into a JavaScript object. This process is called parsing.
In JavaScript, you can use the built-in JSON.parse()
method to convert a JSON string into a JavaScript object. This allows you to access the data and work with it in your application:
// Sample JSON string
const jsonString = `{
"city": "London",
"temperature": 15,
"unit": "Celsius",
"description": "Cloudy",
"forecast": [
{ "day": "Monday", "temp": 16 },
{ "day": "Tuesday", "temp": 18 }
]
}`;
// parse the JSON string into a JavaScript object
const weatherData = JSON.parse(jsonString);
// now we can access the fields just like we would with any JavaScript object
console.log(weatherData.city); // Output: London
For making the GET request, you can use the fetch()
API, which is a modern way to make network requests in the browser. Here's an example of how you might use fetch()
to get the weather data, and process its JSON response:
// Make a GET request to the server to fetch weather data
fetch('/weather')
.then(response => response.json()) // Parse the JSON response
// Note that the response.json() method returns the parsed JSON data
// so we don't have to call JSON.parse() separately in this case
.then(data => handleWeatherResponse(data)) // Call the function to process the data
.catch(error => console.error('Error fetching weather data:', error));
// Function to handle a successful response from the server
// We will process the JSON data here
function handleWeatherResponse(data) {
// Check if the data is valid JSON (optional but good practice)
if (typeof data !== 'object' || data === null) {
console.error('Invalid JSON data received from server');
return;
}
// Extract city name from the response
const city = data.city;
// Extract current temperature and unit
const temperature = data.temperature;
const unit = data.unit;
// Update the user interface with the weather information
document.getElementById('city').textContent = city;
document.getElementById('temperature').textContent = temperature + '°' + unit;
}
Reading JSON Data in Pipedream
Pipedream makes it incredibly easy to work with JSON data. You can receive JSON data from HTTP requests, process it using code steps, and send it to other services or APIs.
When you receive JSON data in a workflow, you can easily inspect and manipulate it using the workflow inspector. The inspector provides a visual representation of the JSON data, making it easy to understand its structure and contents.
For example, we can create an HTTP trigger that listens for incoming requests.
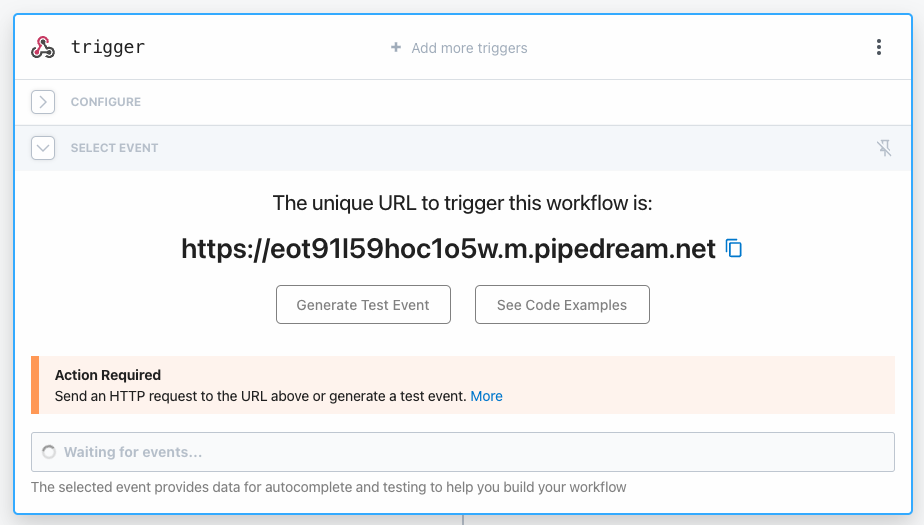
In this case our trigger URL is https://eot91l59hoc1o5w.m.pipedream.net
. Let's send a POST request to this URL with some JSON data, using the curl command:
curl -X POST https://eot91l59hoc1o5w.m.pipedream.net \
-H "Content-Type: application/json" \
-d '{
"city": "London",
"temperature": 15,
"unit": "Celsius",
"description": "Cloudy",
"forecast": [
{ "day": "Monday", "temp": 16 },
{ "day": "Tuesday", "temp": 18 }
]
}'
We can see that the JSON data is received by the workflow, and we can inspect it easily in the UI:
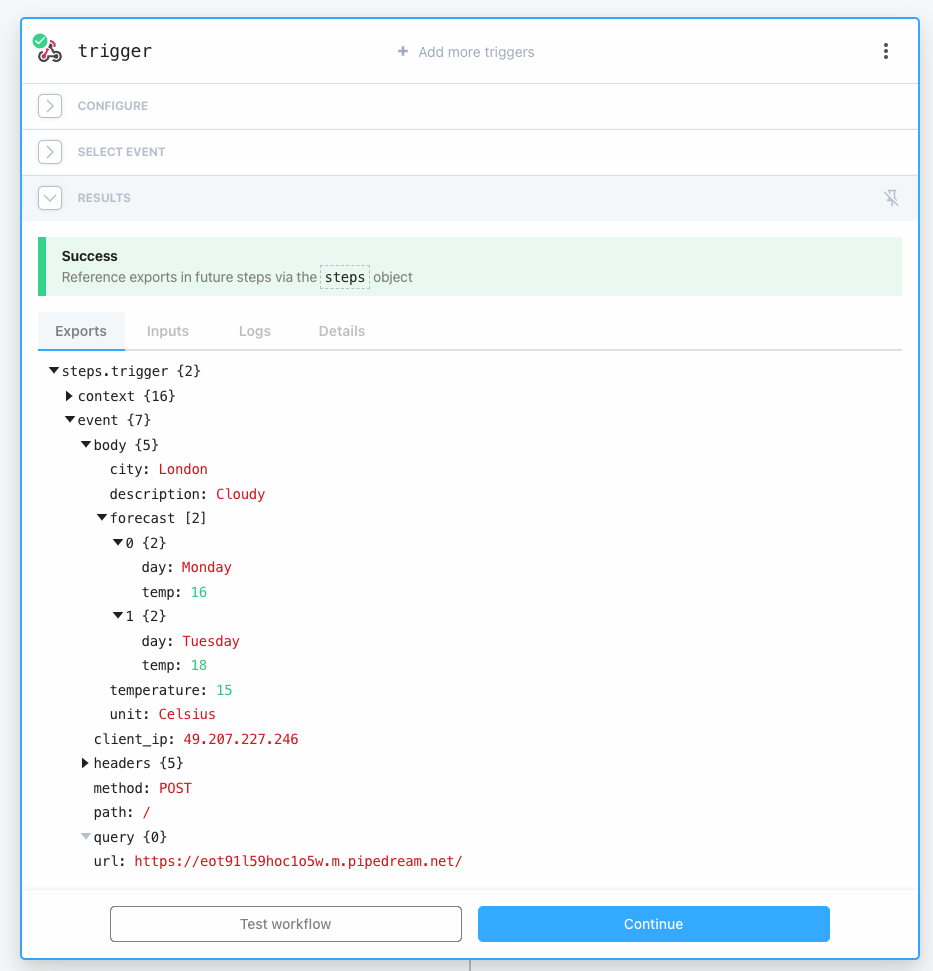
From here, we can select any field within the JSON response and easily pass it to another application. Pipedream has pre-built connections for over 2,000 popular SaaS applications, making it easy to create serverless workflows that pass information between the tools you use every day.