How to send messages to a channel with a Discord Bot
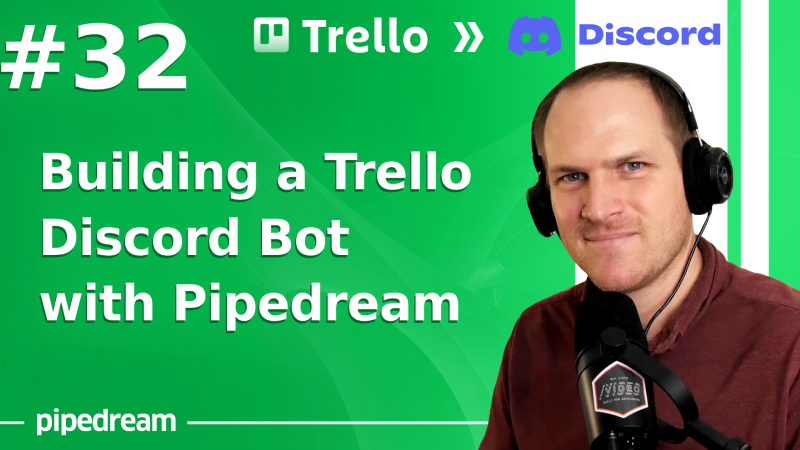
With a Pipedream workflow, you can send messages to Discord with your own Discord Bot.
In this week's Speed Run, we use a new Trello card to trigger the workflow, but this process works with webhooks or any of the thousands of app triggers available in Pipedream.
Set up
If you haven't already, watch the first video to learn how to create and register your Discord Bot with Pipedream:
Sending a message with your Discord Bot
After you've connected your Discord Bot with Pipedream, use the Use Any Discord API in Node.js to scaffold a quick Node.js code step with your Discord Bot's authorization tokens built in.
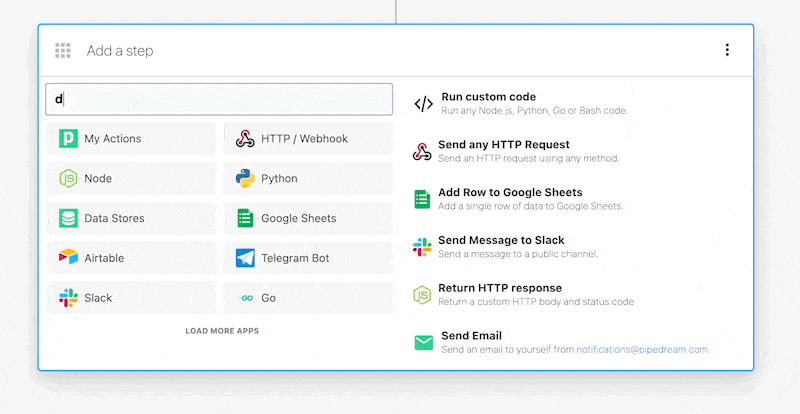
Then you can use the Discord Send Message API endpoint to send a message to your Discord channel:
Below is an example of the basic code in a Node.js code step in Pipedream to send a message to a channel:
import { axios } from "@pipedream/platform"
export default defineComponent({
props: {
discord_bot: {
type: "app",
app: "discord_bot",
}
},
async run({steps, $}) {
return await axios($, {
method: 'POST',
url: `https://discord.com/api/channels/<your channel id here>/messages`,
headers: {
"Authorization": `Bot ${this.discord_bot.$auth.bot_token}`,
},
data: {
embeds: [ steps.generate_embed.$return_value.embed ]
}
})
},
})
Finding your Channel's ID
You may notice there is a channel ID
required in order to send the Discord message to a specific channel.
Here you can make use of the Discord Bot - List Channels action to find all of the channels in your Discord Server.
Select the ID of the channel, and use it in URL of the API request in the code step (labelled <your channel id here>
in the code example above).
Crafting an embed message
For simple messages, you can pass a simple string to the content
payload, but for a richer message, use Discord embeds.
Here's a great free resource to generate Discord embeds.
Then your messages from Pipedream will have images, URLs, fields, and footers. The full reference of available properties are here.
Learn more
Learn more and get connected!
🔨  Start building at https://pipedream.com
📣  Read our blog https://pipedream.com/blog
💬  Join our community https://pipedream.com/community