Adding a Contact Form to Next.js on Vercel
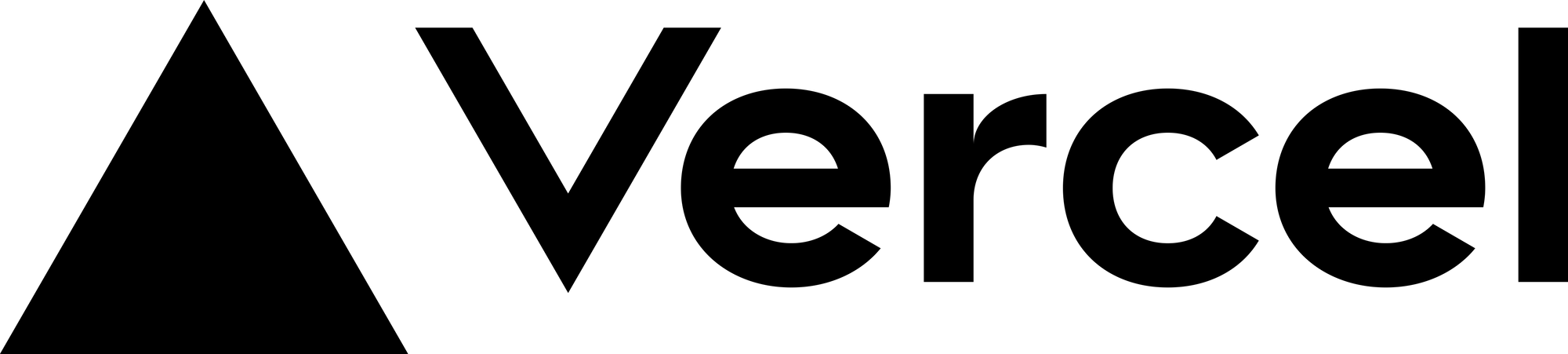
Here's a contact form component you can use in your Next.js project hosted on Vercel - without using a Next.js API route!
We'll use an HTTP request from our Next.js frontend to trigger a Pipedream workflow to notify us on new contact form submissions.
Creating the Backend Workflow
Follow the steps in the video above to create the workflow that's triggered by our ContactForm
component on the frontend, or alternatively just open this link to add it to your Pipedream account:
Contact Form Component
Use this component code below to add a basic contact form to your Next.js site:
ContactForm.js
// components/ContactForm.js
import React, { useState } from "react";
import axios from "axios";
import { useForm } from "react-hook-form";
export default function ContactForm() {
const {
register,
handleSubmit,
formState: { isSubmitting },
} = useForm();
const [successMessage, setSuccessMessage] = useState("");
function onSubmit(data) {
axios
.post("<YOUR PIPEDREAM WORKFLOW URL>", data)
.then((response) => {
setSuccessMessage(
`Thanks for signing up! Check your inbox for updates 😊`
);
})
.catch((e) => console.error(e));
}
return (
<form onSubmit={handleSubmit(onSubmit)}>
<h4>Join our newsletter!</h4>
<input {...register("email")} defaultValue="me@gmail.com"></input>
<button role="submit">{isSubmitting ? "Submitting" : "Submit"}</button>
{successMessage && <p>{successMessage}</p>}
</form>
);
}
And that's it! You now have a functional contact form a statically generated Next.js site.
Learn more and get connected!
🔨  Start building at https://pipedream.com
📣  Read our blog https://pipedream.com/blog
💬  Join our community https://pipedream.com/community