Using Airtable as a Database with Next.js
Airtable and Next.js are like the chocolate and peanut butter of JAMstack. Leverage static site generation and spreadsheet-as-a-db with Pipedream.
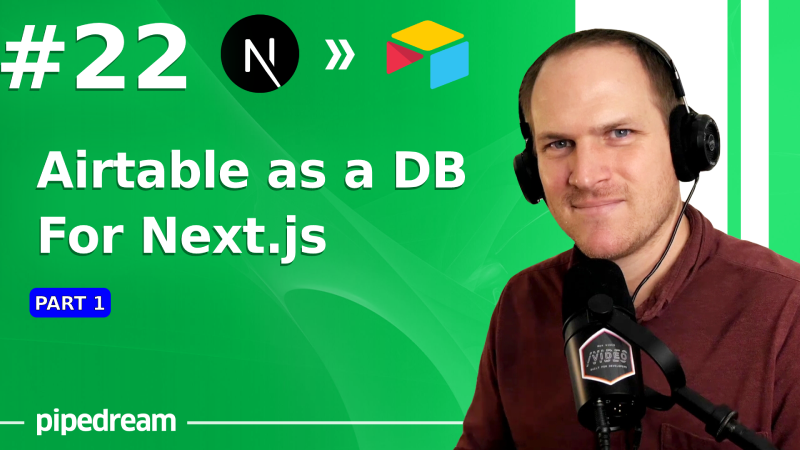
Airtable and Next.js are like the chocolate and peanut butter of JAMstack. You can leverage Airtable as an easy admin view of your data, while leveraging it's API to build static frontend webpages with Next.js.
Watch the video above to see this integration performed live, or continuing reading for text instructions.
But security
Don't forget, your Airtable API key is read and write access to all of your Bases by default.
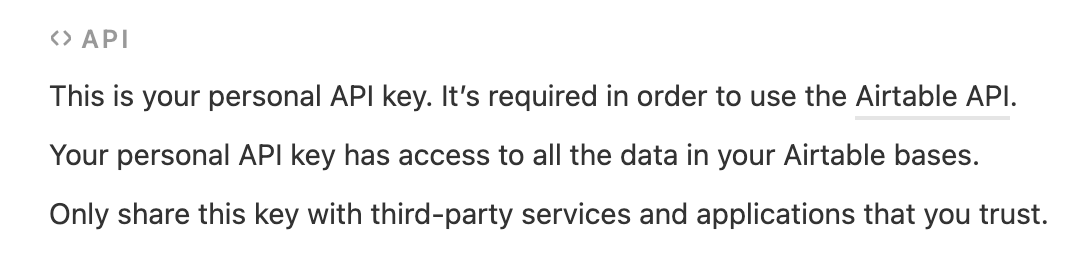
To protect your data from being accessed or manipulated by your website visitors, you'll need to proxy your frontend API calls through a backend service.
You could reach for an Express, Koa or Fastify app. But that means you have to deploy that code somewhere and develop some kind of deployment strategy.
Instead, use a Pipedream workflow to act as a proxy between your Airtable API key and your Next.js app without any code.
Connecting your Airtable account to Pipedream
Connecting your Airtable account to Pipedream is as easy as copying and pasting your Airtable API key from your dashboard.
Then create an new Airtable connection on Pipedream.
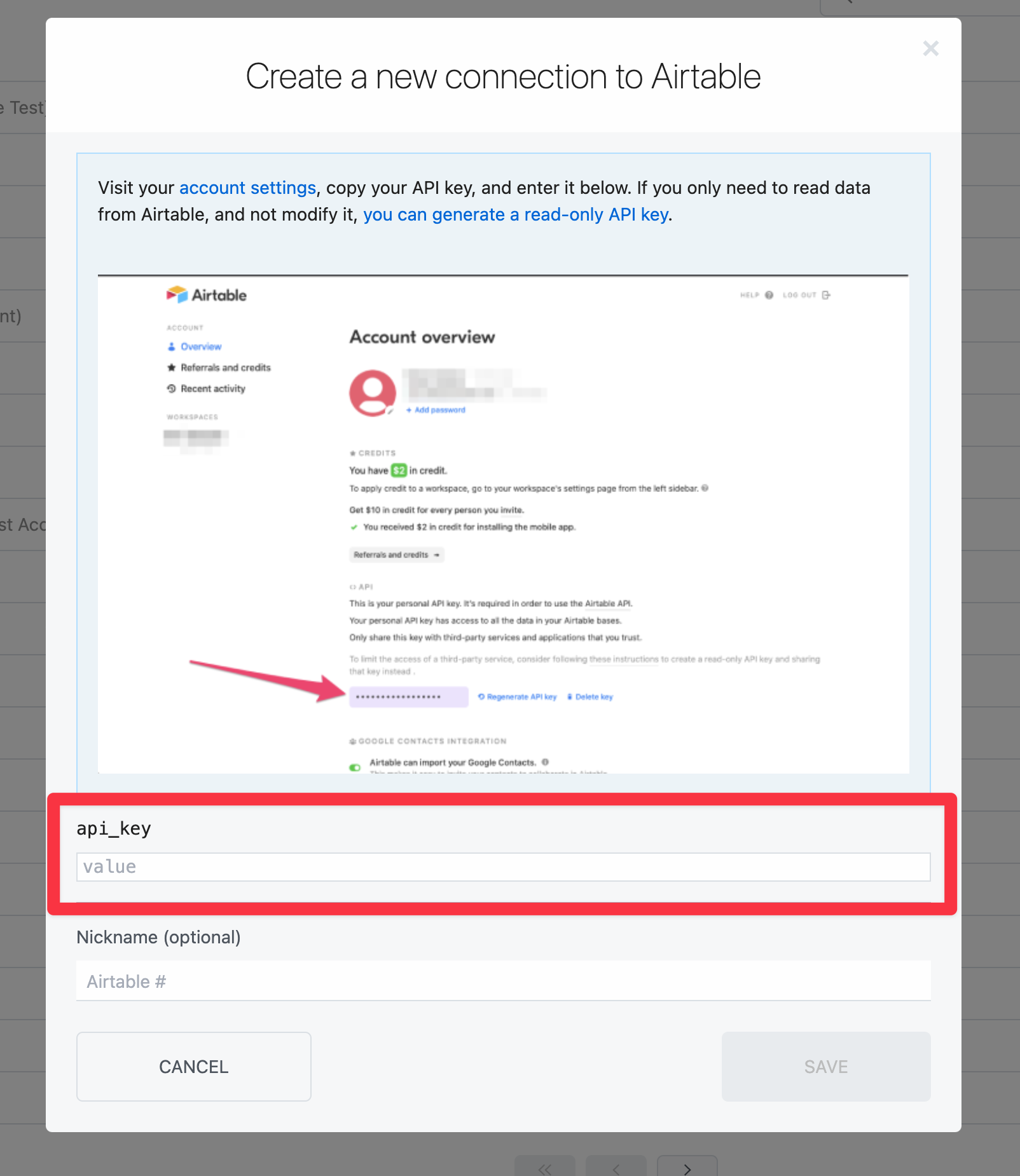
That's it.
Retrieving Records from your Airtable Base
There's already a pre-built action in Pipedream for retrieving records from your Airtable base.
Simply search for List Records under the Airtable app in Pipedream.
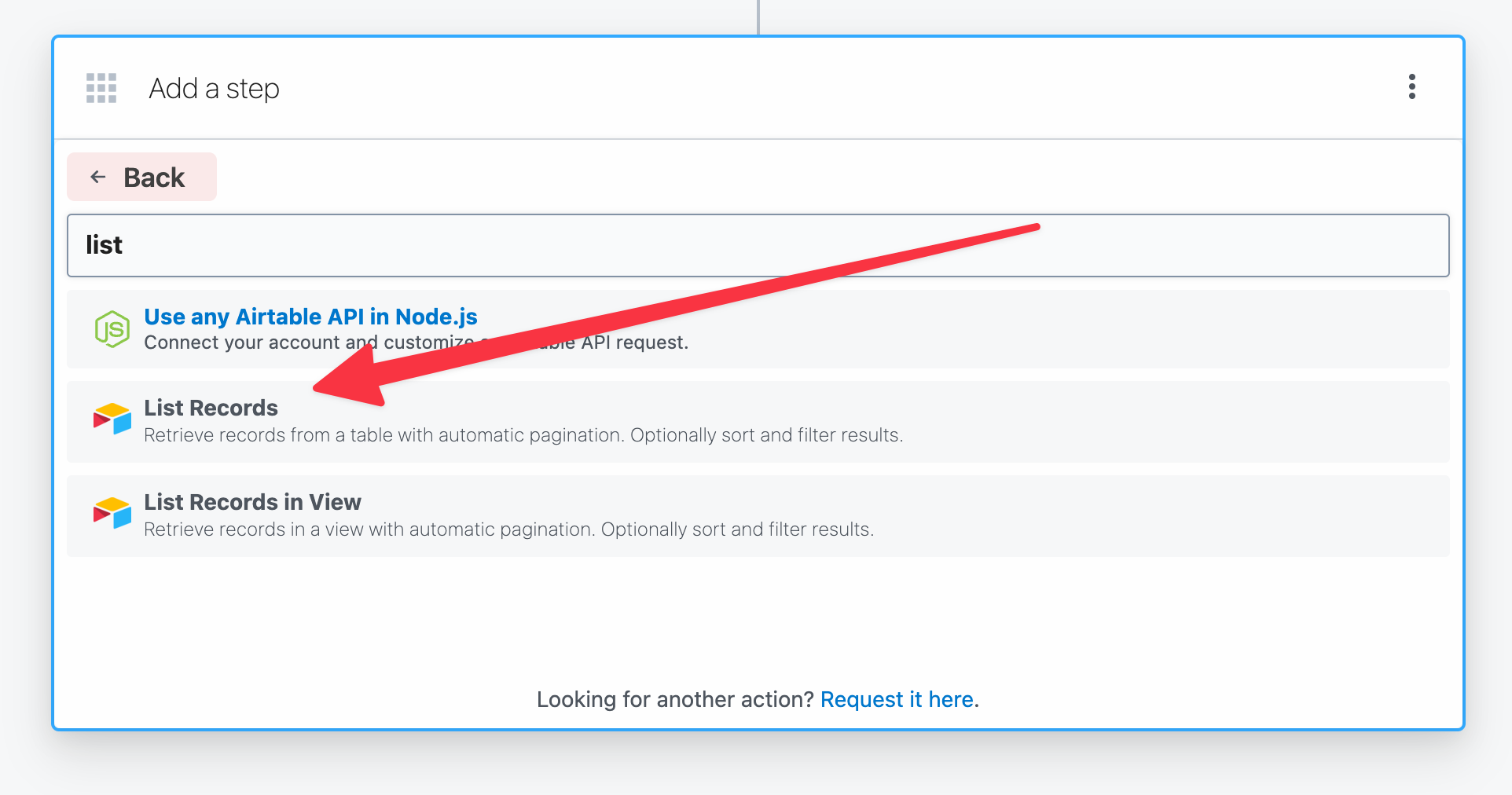
Then you can select your Base and finally Table.
You can even define a filter, sort the records, or add pagination with the pre-built options with a few clicks.
Providing the data to the Next.js app
Now that you have this data, all you have to do is call this URL from your Next.js app:
pages/index.js
export default function Home() {
const [records, setRecords] = useState([]);
async function retrieveRecords() {
const { data } = await axios.get("YOUR PIPEDREAM HTTP ENDPOINT HERE");
setRecords(data);
}
useEffect(() => {
retrieveRecords();
}, []);
return (
<div className="container">
{records.map((record, key) => (
<Record key={key} {...record} />
))}
</div>
);
}
That's it! Full example code here:
https://github.com/PipedreamHQ/nextjs-examples